Smart redirect
Do you have a lot of websites, hosting, or admin panel? And you want all the traffic from many sites got to your relam sites? Then this script will help you in this. Just upload it instead of the main page and all traffic will be directed to your sites. And customization of the script will help you to remove unnecessary traffic.
Script Description
This PHP script is designed to redirect users to a random URL from a given list. The script allows you to customize:
- Exclude users based on language preference.
- Redirect based on device type (mobile or desktop).
- Delay before the redirect is executed.
- A list of URLs for random selection.
The script automatically detects the user’s language and device (mobile or desktop) to perform or skip the redirect according to the settings.
Example configuration:
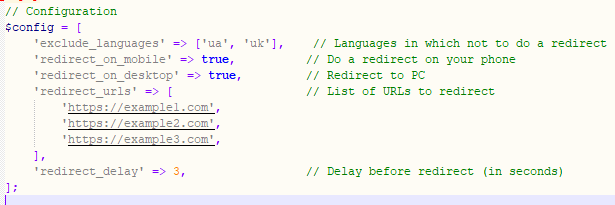
Parameter description:
exclude_languages: List of languages (ISO codes, e.g., ru, en) for which the redirect is not performed.
redirect_on_mobile: Enable/disable redirect for mobile devices (true or false).
redirect_on_desktop: Enable/disable redirect for desktop devices (true or false).
redirect_urls: List of URLs to which a random redirect is performed.
redirect_delay: The delay before the redirect in seconds.
How to use
Preparing the script:
Save the code to a file, such as redirect.php.
Configure the $config array at the beginning of the file according to your requirements.
Placing it on the server:
Upload the redirect.php file to your server in the desired directory.
Integration:
Add a link or customize your website so that users are redirected to redirect.php when they visit certain pages.
Performance Testing:
Open the script in a browser. If the user’s language and device match the settings, it will redirect to a random URL from the list.
Notes
URL list: Make sure the redirect_urls array contains at least one URL. If the array is empty, the script will throw an error.
Delay: Set redirect_delay to 0 if no delay before redirect is required.
Language exceptions: ISO codes for languages can be found here.
If you need to customize or remake this script you can write to the administrator and you for 10$ all will be configured.
Below is the full source code.
<?php
// Configuration
$config = [
'exclude_languages' => ['ua', 'uk'], // Languages in which not to do a redirect
'redirect_on_mobile' => true, // Do a redirect on your phone
'redirect_on_desktop' => true, // Redirect to PC
'redirect_urls' => [ // List of URLs to redirect
'https://example1.com',
'https://example2.com',
'https://example3.com',
],
'redirect_delay' => 3, // Delay before redirect (in seconds)
];
// Function for defining the user language
function getUserLanguage() {
if (!empty($_SERVER['HTTP_ACCEPT_LANGUAGE'])) {
$languages = explode(',', $_SERVER['HTTP_ACCEPT_LANGUAGE']);
return substr($languages[0], 0, 2);
}
return null;
}
// Function for detecting the user's device
function isMobileDevice() {
$userAgent = strtolower($_SERVER['HTTP_USER_AGENT']);
return preg_match('/(iphone|ipod|ipad|android|blackberry|webos|mobile)/i', $userAgent);
}
// Checking conditions for redirect
$userLanguage = getUserLanguage();
$isMobile = isMobileDevice();
if (
(!in_array($userLanguage, $config['exclude_languages'])) && // Language exemption
(($isMobile && $config['redirect_on_mobile']) || // Condition for mobile
(!$isMobile && $config['redirect_on_desktop'])) // PC condition
) {
// Selecting a random URL for redirection
$redirectUrl = $config['redirect_urls'][array_rand($config['redirect_urls'])];
// Delayed redirect
sleep($config['redirect_delay']);
header("Location: $redirectUrl");
exit;
}
// If the redirect is not needed
echo "No redirect needed.";